Java – MyBatis 快查
Mybatis 基本信息
XML配置Mybatis插件
1.引入坐标
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.10</version>
</dependency>
2.创建mybatis-config.xml 配置文件
定义连接数据库和mapper文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- environments表示配置Mybatis的开发环境,可以配置多个环境,在众多具体环境中,使用default属性指定实际运行时使用的环境。default属性的取值是environment标签的id属性的值。 -->
<environments default="development">
<!-- environment表示配置Mybatis的一个具体的环境 -->
<environment id="development">
<!-- Mybatis的内置的事务管理器 -->
<transactionManager type="JDBC"/>
<!-- 配置数据源 -->
<dataSource type="POOLED">
<!-- 建立数据库连接的具体信息 -->
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis-example"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<mappers>
<!-- Mapper注册:指定Mybatis映射文件的具体位置 -->
<!-- mapper标签:配置一个具体的Mapper映射文件 -->
<!-- resource属性:指定Mapper映射文件的实际存储位置,这里需要使用一个以类路径根目录为基准的相对路径 -->
<!-- 对Maven工程的目录结构来说,resources目录下的内容会直接放入类路径,所以这里我们可以以resources目录为基准 -->
<mapper resource="mappers/EmployeeMapper.xml"/>
</mappers>
</configuration>
3.创建一个对应的mapper映射文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!-- namespace等于mapper接口类的全限定名,这样实现对应 -->
<mapper namespace="cn.unsoft.mapper.EmployeeMapper">
<!-- 查询使用 select标签
id = 方法名
resultType = 返回值类型
标签内编写SQL语句
-->
<select id="selectEmployee" resultType="cn.unsoft.pojo.Employee">
<!-- #{empId}代表动态传入的参数,并且进行赋值!后面详细讲解 -->
select emp_id empId,emp_name empName, emp_salary empSalary from
t_emp where emp_id = #{empId}
</select>
</mapper>
Mybatis 与 ibatis 的区别(了解)
MyBatis最初是Apache的一个开源项目iBatis, 2010年6月这个项目由Apache Software Foundation迁移到了Google Code。随着开发团队转投Google Code旗下, iBatis3.x正式更名为MyBatis。代码于2013年11月迁移到Github。
ibatis大概原理
虽然Mybatis与ibatis基本属同一个框架,但是Mybatis和ibatis是有一个区别的,可以看作Mybatis是ibatis的一个更高级的封装和优化
ibatis 是定义在 1.x 版本和 2.x 版本,当时的ibatis其实是一个轻量级的sql框架,其框架的大概原理如下:
上图的通俗解释:
1.其实ibatis里面有一个用来存放sql语句的Map对象,Map对象的key是namespaced的值和crud的id合并,如 namespaced="aaa",crud的id="bbb",则key则为 "aaa.bbb"和“bbb”,它既存namespaced.id也存仅crud的id,而value 则为sql语句。
2.ibatis通过读取ibatis-config.xml文件得知mapper.xml的文件路径,并读取mapper.xml文件。
3.ibatis读取到mapper.xml后,把namespaced和各项crud的id作为key,把自定义sql语句作为value存到Map对象中。
4.ibatis 提供一个封装了Jdbc操作的类SqlSession,由SqlSessionFactory创建,而SqlSession封装了多个操作数据库的方法。
5.当用户创建SqlSession的查询方法时,用户需要提供namespaced和crud的id,ibatis会把用户提供的namespaced和crud的id作为key在它的Map对象中查找到SQL语句,并调用Jdbc进行查询。
用户端执行代码如下:
public void testSelectEmployee() throws IOException {
// 1.创建SqlSessionFactory对象
// ①声明Mybatis全局配置文件的路径
String mybatisConfigFilePath = "mybatis-config.xml";
// ②以输入流的形式加载Mybatis配置文件
InputStream inputStream = Resources.getResourceAsStream(mybatisConfigFilePath);
// ③基于读取Mybatis配置文件的输入流创建SqlSessionFactory对象
SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
// 2.使用SqlSessionFactory对象开启一个会话
SqlSession session = sessionFactory.openSession();
// 3.根据EmployeeMapper接口的Class对象获取Mapper接口类型的对象(动态代理技术)
EmployeeMapper employeeMapper = session.getMapper(EmployeeMapper.class);
// 4. 调用代理类方法既可以触发对应的SQL语句
Employee employee = employeeMapper.selectEmployee(1);
System.out.println("employee = " + employee);
// 4.关闭SqlSession
session.commit(); //提交事务 [DQL不需要,其他需要]
session.close(); //关闭会话
}
ibatis 的缺点
1.用户提供的namespaced和crud的id是以字符串的方式提供,容易出错。
2.SqlSession查询的方法最多只能提供一个对象作为查询条件,如果有多个条件,则需要封装成一个对象传入
3.查询返回的类型是Object的。
因为ibatis的种种缺点,使得Mybatis的诞生
Mybatis 的基本原理
针对ibatis的第一个缺点,Mybatis做了更高的封装,它使用了JDK动态代理技术,对接口进行动态代理生成代理对象,代理对象中所包含的就是以Mapper接口和接口方法名作为namespaced和crud的id.
上图通俗的讲:
1.Mybatis依然使用着ibatis的技术,但不再让用户主动去调用SqlSession的查询方法了,而是要求用户创建一个Mapper接口,利用Mapper接口做JDK的动态代理生成代理对象.
2.通过代理对象可以获得Mapper接口的全类名,也可以获得Mapper接口方法中的方法名,通过这两个组成key值,提交给SqlSession.把用户提供的查询条件参数,自动封装成一个对象并提供给SqlSession.
3.剩下的工作就是ibatis的执行方法了。
4.因此现在明白了,为什么Mapper.xml中的namespaced要与Mapper接口的全类名一致,为什么Mapper.xml的CRUD的id要和Mapper接口中的方法名一致了。
Mybatis 基本配置
Mybatis 基本xml配置详情我们可以在Mybatis 官方中详细查找。
https://mybatis.org/mybatis-3/zh/configuration.html
配置日志输出
在mybatis-config.xml文件中加入配置项;
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
Mybatis 参数传递形式
Mybatis 中有两种参数传递形式,分别为 #{} 和 ${}
#{} 参数传递
使用 #{} 来传递参数,会先使Sql语句中的参数位以 ? 号作为占位符,再使用进行赋值,这样的优点是可以有效的避免了SQL注入的问题,缺点是 #{} 参数传递只能用在查询条件参数传递,不能代替标签、列名、sql关键字等。
${} 参数传递
使用 ${} 参数传递是直接把参米拼接在sql中,不会做占位符操作,缺点是可能导致SQL注入漏洞,优点是可以替代标签、列名、表名、sql关键字等传参。
参数传参
简单类型(单值类型)
单值简单类型是指:传递参数中只有一个参数
说明:单值类型的情况下,传递参数中的参数名可以为随意。
案例:
Employee queryOne(Integer id);
<select id="queryOne" resultType="cn.unsoft.pojo.Employee">
select * from table where id = #{ aaa }
</select>
简单类型(多值类型)
多值简单类型是指:传递参数中有一个以上的参数
说明:多值类型下,既不能使用接口参数的名称,也不能使用随意名称。
方法一:使用 @Param("value") 注解指定参数名。
方法二:使用Mybatis默认的参数 arg0,arg1,arg2....
方法三:使用Mybatis默认的参数 param1,param2,param3...
案例:
Employee queryOne(@Param("a") String name, @Param("b") Integer id);
方案一:
<select id="queryOne" resultType="cn.unsoft.pojo.Employee">
select * from table where name = #{ a } and id = #{ b }
</select>
Employee queryOne(String name, Integer id);
方案二:
<select id="queryOne" resultType="cn.unsoft.pojo.Employee">
select * from table where name = #{ arg0 } and id = #{ arg1 }
</select>
方案三:
<select id="queryOne" resultType="cn.unsoft.pojo.Employee">
select * from table where name = #{ param1 } and id = #{ param2 }
</select>
实体类型(对象类型)
实体对象类型是指:参数传入的是一个对象类。
说明:当传入的是一个对象时,参数名必需与对象中的成员对象一致
Employee {
String empName;
Integer empId;
}
Employee queryByObject(Employee employee);
<select id="queryByObject" resultType="cn.unsoft.pojo.Employee">
select * from table where name=#{empName} and id=#{empId}
</select>
复杂类型(单值类型)
复杂类型是指:使用的是Map等集合类型作为参数。
说明:当传入的是一个Map对象时,参数名必需与Map集合中的key值一致
Map<String,Object> info {
key: "name", value: "xxxx",
key: "id" , value:1
}
Employee queryByObject(Map info);
<select id="queryByObject" resultType="cn.unsoft.pojo.Employee">
select * from table where name = #{name} and id = #{id}
</select>
查询结果输出
输出原则
1.修改、删除、增加这三种操作默认输出 int 类型,无需配置 resultType 标签。
2.查询语句提供以下几种配置 resultType 输出类型
- 对象类型:默认需要填入该pojo对象的全类名,如:
-
<select id="queryByObject" resultType="cn.unsoft.pojo.Employee"> select * from table where name=#{empName} and id=#{empId} </select>
- 官方基本类型:同样默认需要填入官方类型的全类名,如:
-
<select id="queryByObject" resultType="java.lang.String"> select name from table where name=#{empName} and id=#{empId} </select>
默认类别名
Mybatis 对官方基础类型做了72种别名,可以使用别名进行定义返回结果类型:
int 对应 _int
Integer 对应 int
Integer 对应 integer
Map 对应 map
HashMap 对应 hashmap
Double 对应 double
double 对应 _double
等等
详情可查看Mybatis官方文档
https://mybatis.org/mybatis-3/zh/configuration.html#typeAliases
自定义类型别名
对于自定义的对象类型也希望使用别名,我们可以在 mybatis-config.xml 文件中配置别名设置。
方法一:单独设置单个对象的别名:
<settings>
...
</settings>
typeAliases 应配置在 settings 后面
<typeAliases>
<typeAlias alias="Author" type="domain.blog.Author"/>
<typeAlias alias="Blog" type="domain.blog.Blog"/>
<typeAlias alias="Comment" type="domain.blog.Comment"/>
<typeAlias alias="Post" type="domain.blog.Post"/>
<typeAlias alias="Section" type="domain.blog.Section"/>
<typeAlias alias="Tag" type="domain.blog.Tag"/>
</typeAliases>
方法二:使用包设置别名,别名将为该类的类名
<typeAliases>
<package name="domain.blog"/>
</typeAliases>
方法三:希望使用包设置别名的同时,对个别的类做其它别名,在类中单独设置别名,使用 @Alias 注解
@Alias("aaa")
public class Employee { pojo类... }
查询字段名自动映射
对于返回的是自定义对象类型的情况,当我们查询出来的数据库字段与Java对象类型的名称不一致时,我们可以使用数据库字段自动映射驼峰命名法的设置。
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
查询字段名自定义映射
对于数据表中的列字段名和自定义对象中的成员变量名不一致的情况,如果都无法使用自动驼峰命名映射的话,那么可以使用自定义映射 resultMap 标签
其作用是为了处理pojo对象中的成员变量是其它pojo类对象的映射情况,若接收结果的pojo对象中的成员变量存在其它pojo类对象的时候,mybatis无法自动映射,就需要使用自定义映射了。
resultMap 标签是用于声明字段名与pojo对象的成员变量名的之间的映射关系:
<resultMap id="eMap" type="cn.unsoft.pojo.Employee">
<id column="uuid" property="e_uuid"></id>
<result column="name" property="e_name"></result>
</resultMap>
说明:
resultMap 是一个声明自定义映射的标签,它的id将会使用在 CRUD 标签的 resultMap 属性上。
type:映射后最终返回的结果类型
<id>标签:用于定义主键值的映射关系
<result>标签:用于定义非主键估的映射关系
column 属性:声明需要映射的表字段名的名称
property 属性: 声明需要映射到的pojo对象的成员变量名称
定义好 resultMap 映射关系后,就可以在 CRUD 标签中使用 resultMap 映射了
<select id="queryByObject" resultMap="eMap">
select name as 名字 from table where name=#{empName} and id=#{empId}
</select>
返回 Map 数据类型
查询中使用Map接收查询结果数据
在 resultType 中填写 "map"
查询出来的字段名将作为 key,查询出来的列数据将作为 value
<select id="queryByObject" resultType="map">
select name as 名字 from table where name=#{empName} and id=#{empId}
</select>
返回 List 数据类型
查询中若输出多条数据,使用List接收查询结果
在 resultType 中依然填写List的泛型类型即可。
原理:Mybatis 中查询的数据实则是调用 ibatis 中的 selectList 方法查询,所以只需要提供泛型即可。
<select id="queryByList" resultType="employee">
select name from table where id > #{empId}
</select>
插入后返回主键值
在插入数据后我希望把插入的数据自增长值回显
自增长主键值:
<insert id="insert" useGeneratedKeys="true" keyColumn="id" keyProperty="id">
</insert>
useGeneratedKeys: 使用主键回显功能
keyColumn:定义数据库中主键的字段名
keyProperty:定义对象中用于接收主键值的成员变量名
非自增长主键值
方法一:在代码主维护非自增长键值
若非自增长键值是由我们自己生成的并加入到pojo对象中的话,我们就不需要使用mybatis的回显功能了。
employee.setEmpId(111);
employee.setEmpName("aaa");
mapper.insertByEmp(employee);
方法二:在mybatis中维护非自增长键值。
若我们不希望把自增长键值通过自己生成并加入到pojo类,而是希望在xml中定义好自增长值,在插入完成后,由mybatis回显自增长值时,我们可以使用mybatis的 <selectKey>标签,使用方式如下:
<insert id="insertByEmp">
<selectKey order="BEFORE" keyProperty="uuid" resultType="string">
select UUID();
</selectKey>
insert into table (uuid,name) values(#{uuid},#{name})
</insert>
说明:
selectKey 能提供在执行sql语句间加上的查询任务,即执行这条sql之前或之后都会查询
order:定义 sql 语句查询之前执行 selectKey 还是在 sql 语句查询完成之后执行 selectKey.可选 BEFORE 和 AFTER
keyProperty : 指定传入的pojo对象用于存放主键值的成员变量名。
resultType:指定 selectKey 查询出来的值的类型。
原理:mybatis 的 selectKey 查询原理其实是在查询出来后,预先把查询的值赋给pojo对象,然后sql语句中的参数再从pojo对象中获取。
Mybatis 多表查询
多表查询中分为三种情况,分别是 一对一查询、一对多|多对一查询、多对多查询。
一对一查询
一对一查询可以看作A表中的一条记录对应B表中的一条记录。
一对多或多对一查询
一对多或多对一查询是指,A表中的一条记录对应B表中的多条记录,如果把角度站在B表来看,B表的一条记录对应A表的一条记录,可以看作是一对一。
对一举例:一个客户表中对这多个订单表中的订单记录。
实体类案例:
/**
* 客户实体类
*/
@Data
public class Customer {
private Integer customer_id;
private String custom_name;
private Integer order_id;
/**
* 在一对多的情境下
* 客户实体类中存在多个订单类,因此客户实体类中应具条多条订单实体类
*/
private List<Order> orders;
}
订单实体类如下:
/**
* 订单实体类
*/
@Data
public class Order {
private Integer order_id;
private String order_name;
private Integer customer_id;
/**
* 在对一的情境下
* 订单类中只需要包含一个客户实体类的对象即可。
*/
private Customer customer;
}
1.在实例类中,客户实体类应对多个订单实体类,所以在客户实体类中,应当加入计单实体类的List集合。
2.在订单实体类中,因为一个订单只对应一个客户实体类,因此如果要通过查询订单实体类来查询客户信息,在订单实体类中只需要加入实体类对象即可。
resultMap的定义:
1.使用 association 标签来定义对象类型的字段映射
<resultMap id="queryOrderById" type="cn.unsoft.pojo.Order">
<!-- 对于Order表中的普通类型数据的映射 -->
<id column="order_id" property="orderId" />
<result column="order_name" property="orderName" />
<result column="customer_id" property="customerId" />
<!-- 对于Order表中的对象类型映射 -->
<association property="customer" javaType="cn.unsoft.pojo.Customer">
<id column="customer_id" property="customerId" />
<result column="custom_name" property="customName" />
<result column="order_id" property="orderId" />
</association>
</resultMap>
<select id="getOrderById" resultMap="queryOrderById">
select * from t_order o join t_customer c on o.customer_id = c.customer_id
where o.order_id = #{id}
</select>
property:对应在实体类中的对象成员变量的变量名称。
javaType:对应该对象成员变量的实体类类型。
对多举例:查询一个客户中的多条订单信息
1.在客户表中,一个客户记录可以拥有多个订单记录,因此客户实体类中应把订单实体成员变量设为List集合。
resultMap 的定义:
1.使用 collection 定义一对多条实体类的情况
<resultMap id="queryCustomer" type="cn.unsoft.pojo.Customer">
<!-- 普通类型的字段映射 -->
<id column="customer_id" property="customerId" />
<result column="custom_name" property="customName"/>
<result column="order_id" property="orderId"/>
<!-- 对多时的实体类对象的字段映射 -->
<collection property="orders" ofType="cn.unsoft.pojo.Order">
<id column="order_id" property="orderId"/>
<result column="order_name" property="orderName"/>
<result column="customer_id" property="customerId"/>
</collection>
</resultMap>
<select id="queryList" resultMap="queryCustomer">
select * from t_order o join t_customer c
on o.customer_id = c.customer_id
</select>
property:该实体类在成员变量中的变量名称。
ofType:实体类集合的泛型类型。
注意:collection 使用的是ofType定义泛型类型,不是 association 中的 javaType
开启深层自动注入数据功能
Mybatis 中还可以对深层次的对象做自动注入功能。
在设置中加入自动映射功能:
<settings>
<!-- 开启日志功能 -->
<setting name="logImpl" value="STDOUT_LOGGING"/>
<!-- 开启驼峰映射功能 -->
<setting name="mapUnderscoreToCamelCase" value="true"/>
<!-- 开启深层自动映射功能 -->
<setting name="autoMappingBehavior" value="FULL"/>
</settings>
autoMappingBehavior 功能有三种可选项:
NONE:不对字段进行自动注入数据
PARTIAL:只对第一层字段进行自动注入数据,对于成员变量是其它pojo对象不会进行注入
FULL:对所有成员变量进行自动注入数据,但前提是在 resultMap 中指定了成员变量类型
要让Mybatis实现深层注入数据,需要声明 id 标签项,举例
<resultMap id="queryCustomer" type="cn.unsoft.pojo.Customer">
<!-- 普通类型的字段映射 -->
<id column="customer_id" property="customerId" />
<result column="custom_name" property="customName"/>
<result column="order_id" property="orderId"/>
<!-- 对多时的实体类对象的字段映射 -->
<collection property="orders" ofType="cn.unsoft.pojo.Order">
<id column="order_id" property="orderId"/>
<result column="order_name" property="orderName"/>
<result column="customer_id" property="customerId"/>
</collection>
</resultMap>
<select id="queryList" resultMap="queryCustomer">
select * from t_order o join t_customer c
on o.customer_id = c.customer_id
</select>
开启自动深层注入数据后,至少要设置 <id> 标签,这样 <result> 标签就可以不需要定义了,Mybatis 会自动根据已有的resultMap定义,自动识别并注入数据,当然前提是字段名和成员变量名需要驼峰后一一对应。
Mybatis 动态标签
if 和 where 标签
<if> 可以判断传入的参数,最终是否添加到语句中
test 属性 内部做比较运算,最终得出 true 时将标签内的sql语句进行拼接,false 不拼接标签内部语句
test 属性可用的比较符号为:and 和 or
大于和小于不推荐直接写符号,应写 > 和 <
<where> 标签通常和 <if> 标签一同使用,因为<if> 有以下几种情况有可能出现sql语句拼接错误
如下代码:
<select id="query" resultType="int">
select * from table
where
<if test="id != null">
id = #{id}
</if>
<if test="name != null">
and name = #{name}
</if>
</select>
当 id = null 时:将会出现 where and name = xx 的情况,会出现sql拼接错误
当 id = null 且 name = null 时:将会出现 select * from table where 的情况,会出现sql拼接错误
因此我们需要与 <where> 配合使用
<where> 标签的作用有两个:
1.自动添加 where 关键字,where 内部有任何一个if满足,自动添加 where 关键字,不满足去掉 where
2.自动去掉多余的 and 和 or 关键字
set 标签
set 标签是用于 update 时,和where 标签类似,当存在参数数据时则对该参数进行 set 更新数据,与 if 标签合并使用
set 标签的作用:
1.自动去除多余的逗号
2.自动添加 set 关键字
<update id="update">
update table
<set>
<if test="name != null">
name = #{name}
</if>
<if test="salary != null">
salary = #{salary}
</if>
</set>
</update>
trim 标签(了解)
使用trim标签控制条件部分两端是否包含某些字符
- prefix属性:指定要动态添加的前缀
- suffix属性:指定要动态添加的后缀
- prefixOverrides属性:指定要动态去掉的前缀,使用“|”分隔有可能的多个值
- suffixOverrides属性:指定要动态去掉的后缀,使用“|”分隔有可能的多个值
!-- List<Employee> selectEmployeeByConditionByTrim(Employee employee) -->
<select id="selectEmployeeByConditionByTrim" resultType="com.atguigu.mybatis.entity.Employee">
select emp_id,emp_name,emp_age,emp_salary,emp_gender
from t_emp
<!-- prefix属性指定要动态添加的前缀 -->
<!-- suffix属性指定要动态添加的后缀 -->
<!-- prefixOverrides属性指定要动态去掉的前缀,使用“|”分隔有可能的多个值 -->
<!-- suffixOverrides属性指定要动态去掉的后缀,使用“|”分隔有可能的多个值 -->
<!-- 当前例子用where标签实现更简洁,但是trim标签更灵活,可以用在任何有需要的地方 -->
<trim prefix="where" suffixOverrides="and|or">
<if test="empName != null">
emp_name=#{empName} and
</if>
<if test="empSalary > 3000">
emp_salary>#{empSalary} and
</if>
<if test="empAge <= 20">
emp_age=#{empAge} or
</if>
<if test="empGender=='male'">
emp_gender=#{empGender}
</if>
</trim>
</select>
choose 、when、otherwise标签
choose 标签就好比 Java 中的 switch..case 的方法。
choose 中输入多个条件,但最终只会选择其一。
<select id="choose" resultType="int">
select * from table
where
<choose>
<!-- 当name不等于null时,只拼接name,不拼接salary -->
<when test="name != null">name = #{name}</when>
<!-- 当salary不等于null时,只拼接salary,不拼接name -->
<when test="salary != null">salary = #{salary}</when>
<!-- 当name和salary都为null时,只拼接 1=1 即最终都没有可选时,会使用otherwise-->
<otherwise>1=1</otherwise>
</choose>
</select>
foreach 标签
foreach 是可以用于批量生成sql片段的标签,可以做一些数组遍历生成sql片段
<select id="selectList" resultType="int">
select * from table
where
id in
<foreach collection="employeeList" open="(" close=")" separator="," item="employee">
#{employee.id}
</foreach>
</select>
collection:接收的用于循环遍历的列表参数值
open:开始遍历前增加的文本
close:结束遍历后增加的文本
separator:每一次遍历后用于分隔的文本
item:每一次遍历的值的变量名
使用foreach同时执行多条sql语句
<update id="multiUpdate">
<foreach collection="employeeList" item="emp">
update employee set
emp_id = #{emp.empID},
emp_name = #{emp.name}
where emp_id = #{emp.empId};
</foreach>
</update>
注意:数据库有可能不支持一次执行中执行多条sql语句的情况,我们可以在连接mysql的url上增加一个参数以开启支持
jdbc:mysql://localhost:3306/mybatis-example?allowMultiQueries=true
sql片段标签
用于定义一些sql片段的标签,可以提取出来复用在其它CRUD标签中。
<sql id="sqls">
select * from
</sql>
<select id="selectFromSQL" resultType="int">
<include refid="sqls" />
table where id = 1
</select>
PageHelper分页插件使用
1.引入坐标
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.2.0</version>
</dependency>
2.把分页插件设置到mybatis中
<settings>
...
</settings>
<plugins>
<plugin interceptor="com.github.pagehelper.PageInterceptor">
<property name="helperDialect" value="mysql"/>
</plugin>
</plugins>
3.使用分页查询
PageHelper.startPage(1,2);
List<Employee> list = mapper.queryAll();
PageInfo<Employee> pageInfo = new PageInfo<>(list);
pageInfo.getTotal();
pageInfo.getList();
pageInfo.getPages();
pageInfo.getPageNum();
pageInfo.getPageSize();
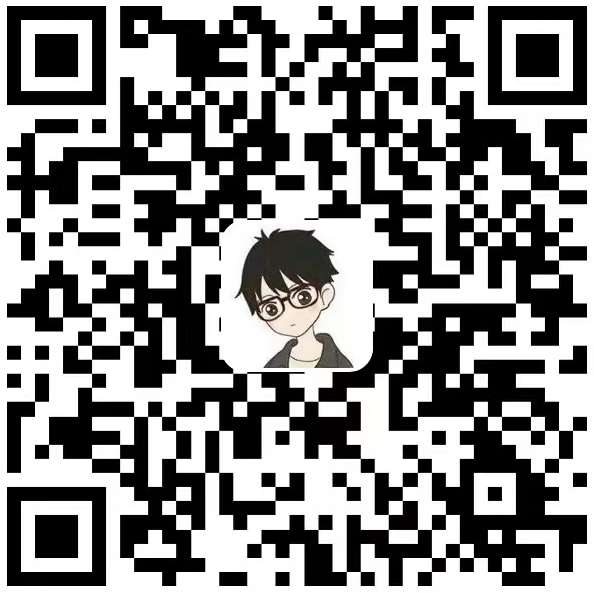
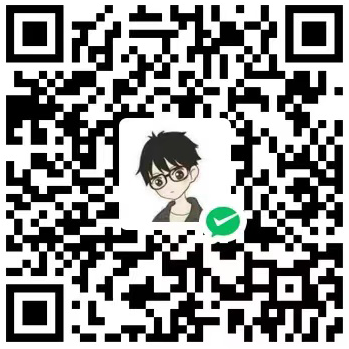
共有 0 条评论